學習基本的 javascript 和 node.js
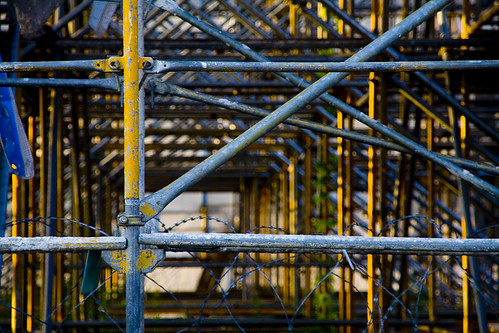
安裝完 node.js 開發環境並且也知道了常用的 npm
指令之後, 接下來來看一些 javascript 以及 node.js 的基礎. 俗話說萬丈高樓平地起, 這些基本概念沒學好之後可是很難精進的.
Examples and source
範例檔案請見 github
執行 javascript 檔案
我們來寫一個可以在 terminal 列出 Hey you
的 javascript 程式. 打開你的文字編輯器然後輸入 console.log( 'Hey you' );
並另存新檔成 hey.js
到桌面. 然後打開 terminal 輸入以下指令.
$ cd ~/Desktop $ node hey.js # -> Hey you
使用外部檔案
要寫出模組化的程式就必須把程式切分的乾淨. node.js 遵照 CommonJS 的慣例, 用 require
以及 exports
來作檔案和模組之間的溝通.
從實際範例會比較清楚的看到該如何使用. 假設我們要從 source.txt
這個檔案讀取資料並在 terminal 印出. 之後再將裡面的 I am
改成 You are
並另存新檔為 changed.txt
. 在這裡我們故意將上面所有的動作分成三個檔案 read.js
, write.js
還有 run.js
, 並將這四個檔案放在同一個資料夾裡面. 最後執行 run.js
來得到我們要的結果.
source.txt
I am Ben.
read.js | 這裡負責讀檔以及在 terminal 列出
// require core module `file system` var fs = require( 'fs' ); // exports 2 methods for other modules or files to use module.exports = { read : function( path, callback ){ // read file data synchronizely var data = fs.readFileSync( path ); // execute the callback if there is one callback && callback( data.toString()); }, print : function( data ){ // print out the data console.log( data ); } };
write.js | 我們將寫入的部分放進這個檔案
// require core module `file system` var fs = require( 'fs' ); // exports 1 method for other modules or files to use module.exports = { write : function( filename, data ){ // write to file synchronizely fs.writeFileSync( filename, data ); } };
run.js | 執行檔
// to use methods from other files we simply use `require` with path name var reader = require( './read' ), writer = require( './write' ); // call `read` method from read.js to read `source.txt` reader.read( './source.txt', function( data ){ // change `I am` to `You are` var changed = data.replace( 'I am', 'You are' ); // print out the data reader.print( data ); // save the changes to `changed.txt` writer.write( './changed.txt', changed ); });
Q&A
下面 3 種語法有什麼不同呢?
var something = require( './something' ); var something = require( './something.js' ); var something = require( 'something' );
第一種和第二種作法基本上一樣. 如果 node.js 找不到 ./something
這個檔案便會自動尋找 ./something.js
. 所以基本上用 ./something.js
會快一點點. 至於第三種呢, node.js 會尋找叫做 something
的套件. 關於如何寫 npm 套件會在後面的文章另外說明.
exports VS module.exports
他們很相似但是還是有些不同. 底下兩種寫法用起來一樣.
用 `module.exports`
module.exports = { do_a : function(){ // do something ... }, do_b : function(){ // do something ... } };
用 `exports`
exports.do_a = function(){ // do something ... }; exports.do_b = function(){ // do something ... };
以上兩者我們都可以像下面這樣使用
var something = require( './something' ); something.do_a(); something.so_b();
但有些時候我們只想要 require 後直接使用, 用上面的作法的話我們必須要
var something = require( './something' ); something.something();
這樣實在有點煩人, 我們必須重複 module 和 method 的名稱都為 something
. 但要是我們不想重複呢?
var something = require( './something' ); something();
這就是 exports
和 module.exports
不同的地方. module.exports
你可以直接像下面那樣用但是 exports
不行.
// this works module.exports = function(){ // do something }; // this is not going to work exports = function(){ // do something };
require
只會載入檔案一次然後存放在記憶體裡面, 所以不用怕效能的問題.
下面是一些從其他語言過來的人比較常對 javascript 搞混的幾點
用 node.js 以及 mongoDB 來作 C.R.U.D
學完 javascript 以及 node.js 的基本. 接下來我們來用 node.js 以及 mongoDB 寫一個 http server 來作個有 C.R.U.D( create, read, update, delete data ) 動作的網站. TODO list 會是一個不錯的目標.