Learning the basics of javascript and node.js
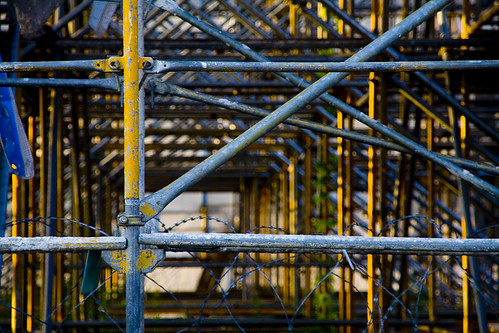
After setting up the node.js development environment and know some common uses of npm
commands. It’s time to learn the basics of javascript and node.js.
Examples and source
Examples and source are available on github
Execute javascript files
Let’s write a simple javascript to print Hey you
on terminal. First open your text editor or IDE, type console.log( 'Hey you' );
and save it as hey.js
on your desktop. Then open your terminal and type the following commands.
$ cd ~/Desktop $ node hey.js # -> Hey you
Use external files
To write a more modular application, you must split your code into files and break them into modules. node.js follows CommonJS conventions. It uses require
and exports
to communicate between files and modules.
It’s easier to see how it works from examples. Say we need to read data in the file source.txt
and print it out. After that we change all I am
to You are
and save it as a new file named changed.txt
. Instead writing all these in a single file we break them into 3 files called read.js
, write.js
and run.js
. All these 4 files are in the same directory and we only want to execute run.js
to get all the above working.
source.txt
I am Ben.
read.js | Read the source file and print to the terminal
// require core module `file system` var fs = require( 'fs' ); // exports 2 methods for other modules or files to use module.exports = { read : function( path, callback ){ // read file data synchronizely var data = fs.readFileSync( path ); // execute the callback if there is one callback && callback( data.toString()); }, print : function( data ){ // print out the data console.log( data ); } };
write.js | Split the write action to this file
// require core module `file system` var fs = require( 'fs' ); // exports 1 method for other modules or files to use module.exports = { write : function( filename, data ){ // write to file synchronizely fs.writeFileSync( filename, data ); } };
run.js | Flow control
// to use methods from other files we simply use `require` with path name var reader = require( './read' ), writer = require( './write' ); // call `read` method from read.js to read `source.txt` reader.read( './source.txt', function( data ){ // change `I am` to `You are` var changed = data.replace( 'I am', 'You are' ); // print out the data reader.print( data ); // save the changes to `changed.txt` writer.write( './changed.txt', changed ); });
Q&A
So what’s the differences between the following 3?
var something = require( './something' ); var something = require( './something.js' ); var something = require( 'something' );
The first and second are basically the same. If node.js can’t find the file ./something
it automatically looks for ./something.js
. In other words, with ./something.js
it loads a bit faster. As for the 3rd one. node.js looks for the package something
not the file. We will talk about this later on the npm
parts.
exports VS module.exports
They are similar but different. The following two works the same.
Using `module.exports`
module.exports = { do_a : function(){ // do something ... }, do_b : function(){ // do something ... } };
Using `exports`
exports.do_a = function(){ // do something ... }; exports.do_b = function(){ // do something ... };
We can use both in another file with
var something = require( './something' ); something.do_a(); something.so_b();
However sometimes we just want to require something and use it, with the above example we have to it do like
var something = require( './something' ); something.something();
It’s kind of annoying that we have to repeat something
for both module and method name. What if we just want to do
var something = require( './something' ); something();
That’s the difference between exports
and module.exports
. with module.exports
you can use it like the following but with exports
you can’t.
// this works module.exports = function(){ // do something }; // this is not going to work exports = function(){ // do something };
require
only loads the file once and will store them in the memory, so don’t be afraid to use it.
The followings are some basics of javascript that people from another language might not be familiar with.
C.R.U.D with node.js and mongoDB
We have gone through the basics of javascript and node.js. Next we are going to create a http server using node.js and mongoDB as our data store to build a simple C.R.U.D( create, read, update, delete data ) website. A TODO list is a great practice to try!