A jQuery plugin that Make your Javascript application more flexible
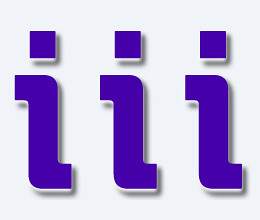
Description
Javascript is an event-driven programming language. Therefore with heavy frontend applications your code is easy to have nested callbacks. And this make your code less flexible.
With jQuery Queue plugin you can add functions in a queue outside the function scope; execute the queued functions later. It makes your code easily to be more modularize. It is a must have if you write heavy frontend websites.
Demo
- Click here to see the demo
- The demo page is also included in the source file
Download
- Download source code from Github
Requires
- jQuery 1.3.0+
Browser Compatibility
- Firefox 2.0+
- Internet Explorer 6+
- Safari 3+
- Opera 10.6+
- Chrome 8+
Installation
- First, make sure you are using valid DOCTYPE
- Include nessesary JS files
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.5.1/jquery.min.js"></script> <script type="text/javascript" src="path-to-file/jquery.queue.js"></script>
Usage
Syntax
Push function to a queue
$.queue( 'add', 'queueName', function( arg1, arg2, arg3 ){ // do something here });
Remove function from a queue
var someFunction = function( arg1, arg2, arg3 ){ // some code } $.queue( 'remove', 'queueName', 'someFunction' );
Call queue functions
$.queue( 'call', 'queueName', [ arg1, arg2, arg3 ]); // or $.queue( 'call', 'queueName', arg );
Clear queue
$.queue( 'clear', 'queueName' );
Example code
Push function ‘Store ajax call data’ to ‘afterLoadPhotos’ queue, here we use $.secret plugin
// store data outside a ajax call $.queue( 'in', 'afterLoadPhotos', function( total, photos ){ // store( cache ) flickr photo data for later use $.secret( 'in', 'totalPhotos', total ). secret( 'in', 'photos', photos ); });
Push function ‘append to DOM’ to ‘afterLoadPhotos’ queue
// append photos to the dom using data 'outside' the ajax call $.queue( 'add', 'afterLoadPhotos', function( total, photos ){ var fragment = ''; $.each( photos, function( key, photo ){ fragment = fragment + '<li><a href="' + photo.imagePath + '">' + '<img scr="' + photo.thumb_path + '"/><span>' + photo.title + '</span></a></li>'; }); $( '#gallery' ).append( fragment ); });
Execute queue functions for ‘afterLoadPhotos’
$.ajax({ type : 'get', url : '/flickr/photos', dataType : 'json', success : function( rsp ){ $.queue( 'call', 'afterLoadPhotos', [ rsp.total, rsp.photos ]); } });
With this plugin we don’t have to defined what we are going to do after the ajax call or inside the ajax call. Not only with ajax call, we can also use it to connect different class and module more flexible through out the whole application.
Namespace
$.queue
supports 1 layer namespace. With large application we might need to split our code into modules.
Example code:
// push a function to getPhotos queue under FLICKR module $.queue( 'add', 'FLICKR.afterGetPhotos', function( secret, extraParams ){ // do stuffs here }); // another function for searching images on google $.queue( 'add', 'GOOGLE.searchImage', function( args ){ // do other stuffs here }